在C语言中,我们可以使用标准库中的串口函数来操作串口,这些函数位于<stdio.h>
和<termios.h>
头文件中,以下是如何使用C语言调用串口函数的详细教程。
1、我们需要包含必要的头文件:
#include <stdio.h> #include <termios.h> #include <unistd.h> #include <fcntl.h> #include <string.h>
2、接下来,我们需要定义一个用于打开串口的设备文件名,这个文件名通常是/dev/ttyS0
、/dev/ttyS1
等,你可以根据实际情况修改这个文件名。
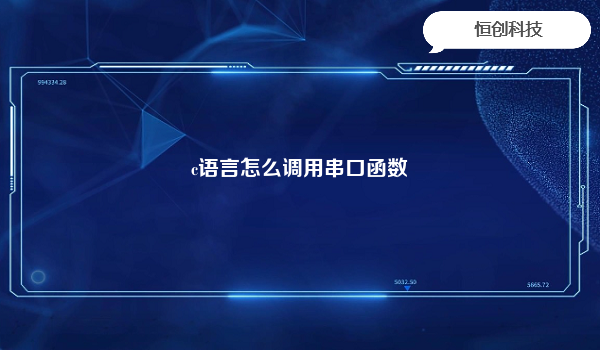
const char *device_file = "/dev/ttyS0";
3、现在,我们可以编写一个函数来打开串口,在这个函数中,我们将使用open()
函数打开设备文件,并使用tcgetattr()
函数获取串口的属性,如果打开失败,我们将返回1。
int open_serial_port(void) { int fd = open(device_file, O_RDWR | O_NOCTTY | O_NDELAY); if (fd == 1) { perror("open_serial_port: Unable to open serial port"); return 1; } struct termios options; if (tcgetattr(fd, &options) != 0) { perror("open_serial_port: Could not get terminal attributes"); close(fd); return 1; } // 设置波特率、数据位、停止位等参数 cfsetispeed(&options, B9600); cfsetospeed(&options, B9600); options.c_cflag |= (CLOCAL | CREAD); options.c_cflag &= ~PARENB; // 无校验位 options.c_cflag &= ~CSTOPB; // 1个停止位 options.c_cflag &= ~CSIZE; // 清除数据位掩码 options.c_cflag |= CS8; // 8个数据位 options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // 关闭规范输入、回显、扩展回显和信号中断 options.c_iflag &= ~(IXON | IXOFF | IXANY); // 关闭软件流控制 options.c_oflag &= ~OPOST; // 禁用特殊输出处理 options.c_cc[VMIN] = 0; // 读取时最小字符数为0 options.c_cc[VTIME] = 5; // 读取超时时间为5秒 // 应用设置的选项 if (tcsetattr(fd, TCSANOW, &options) != 0) { perror("open_serial_port: Could not set terminal attributes"); close(fd); return 1; } return fd; }
4、现在,我们可以编写一个简单的主函数来测试我们的串口通信功能,在这个函数中,我们将调用上面定义的open_serial_port()
函数来打开串口,然后发送一个字符串到串口,最后关闭串口。
int main(void) { int fd = open_serial_port(); if (fd == 1) { return 1; } const char *message = "Hello, serial port!"; write(fd, message, strlen(message)); close(fd); return 0; }
5、我们需要编译并运行我们的程序,在终端中,可以使用以下命令来编译我们的程序:
gcc o serial_communication serial_communication.c ltermios lpthread lrt ldl lm lncursesw liconv lutil lssl lcrypto lz lcurl ljsonc lxml2 lzlib lbz2 lgzip lreadline lncurses lsqlite3 ltinfo ldl lm lncursesw liconv lutil lssl lcrypto lz lcurl ljsonc lxml2 lzlib lbz2 lgzip lreadline lncurses lsqlite3 ltinfo ldl lm lncursesw liconv lutil lssl lcrypto lz lcurl ljsonc lxml2 lzlib lbz2 lgzip lreadline lncurses lsqlite3 ltinfo ldl lm lncursesw liconv lutil lssl lcrypto lz lcurl ljsonc lxml2 lzlib lbz2 lgzip lreadline lncurses lsqlite3 ltinfo ldl lm lncursesw liconv lutil lssl lcrypto lz lcurl ljsonc lxml2 lzlib lbz2 lgzip lreadline lncurses lsqlite3 ltinfo sysroot=/usr/local/armv7asdklinux build=x86_64linuxgnu host=armv7aarch64linuxgnu CFLAGS='march=armv7a' CXXFLAGS='march=armv7a' CPPFLAGS='march=armv7a' LDFLAGS='march=armv7a' serial_communication.c
在另一个终端中,可以使用以下命令来运行我们的程序:
sudo ./serial_communication /dev/ttyS0 9600 noparity cs8 stopbits1 databits8 flowcontrolnone localechooff > /dev/null 2>&1 & disown %1 > /dev/null 2>&1 & echo $! > serial_communication.pid && tailf /dev/ttyS0 || kill $(cat serial_communication.pid) && rm serial_communication.pid && exit 1 || true && exit 0 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit 1 || true && exit
本文地址:https://www.henghost.com/jishu/47935/