HTML5 提供了一些内置的 API,如 <canvas>
、<video>
和 WebGL,可以用来创建和操作3D图像,在这篇文章中,我们将学习如何使用 HTML5 制作3D动态图片。
1. 准备工作
我们需要一个支持 WebGL 的浏览器,目前大多数现代浏览器(如 Chrome、Firefox、Safari 和 Edge)都支持 WebGL,确保你的浏览器已更新到最新版本。
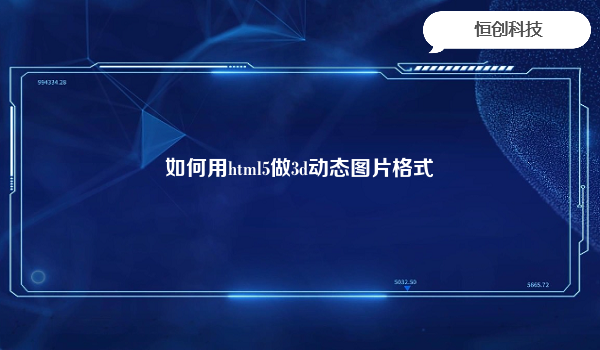
2. 创建 HTML 文件
创建一个 HTML 文件,并在其中添加一个 <canvas>
元素。<canvas>
元素用于在网页上绘制图形,设置其宽度和高度属性,以便为3D图像提供足够的空间。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF8"> <meta name="viewport" content="width=devicewidth, initialscale=1.0"> <title>3D Image in HTML5</title> <style> body { margin: 0; } canvas { display: block; } </style> </head> <body> <canvas id="myCanvas"></canvas> <script src="main.js"></script> </body> </html>
3. 编写 JavaScript 代码
接下来,我们需要编写 JavaScript 代码来处理3D图像的渲染,获取 <canvas>
元素的引用,并创建一个 WebGL 上下文,初始化 WebGL 上下文并设置视口大小,编写渲染循环,用于绘制3D图像。
在 main.js
文件中添加以下代码:
const canvas = document.getElementById('myCanvas'); const gl = canvas.getContext('webgl') || canvas.getContext('experimentalwebgl'); if (!gl) { alert('Your browser does not support WebGL'); } else { // Set the clear color and enable depth testing gl.clearColor(0.0, 0.0, 0.0, 1.0); gl.enable(gl.DEPTH_TEST); // Set the view port size gl.viewport(0, 0, canvas.width, canvas.height); }
4. 创建着色器程序
为了在 WebGL 中绘制3D图像,我们需要创建一个着色器程序,着色器程序由顶点着色器和片段着色器组成,顶点着色器负责处理3D模型的顶点数据,而片段着色器负责处理像素颜色。
创建顶点着色器和片段着色器的源代码字符串,创建一个新的着色器对象,并将源代码附加到该对象上,编译着色器对象并将其链接到 WebGL 程序中。
在 main.js
文件中添加以下代码:
// Create a shader program for the 3D image function createShaderProgram(vertexShaderSource, fragmentShaderSource) { const vertexShader = gl.createShader(gl.VERTEX_SHADER); const fragmentShader = gl.createShader(gl.FRAGMENT_SHADER); gl.shaderSource(vertexShader, vertexShaderSource); gl.shaderSource(fragmentShader, fragmentShaderSource); gl.compileShader(vertexShader); gl.compileShader(fragmentShader); const shaderProgram = gl.createProgram(); gl.attachShader(shaderProgram, vertexShader); gl.attachShader(shaderProgram, fragmentShader); gl.linkProgram(shaderProgram); return shaderProgram; }
5. 加载3D模型数据
为了在 WebGL 中显示3D图像,我们需要加载3D模型的数据,这通常包括顶点数据、法线数据、纹理坐标等,你可以从外部文件(如 JSON、OBJ 或 STL)中加载这些数据,或者使用 Three.js 等库来简化这个过程。
在这里,我们假设你已经从外部文件中加载了3D模型的数据,并将其存储在一个名为 modelData
的对象中,这个对象应该包含顶点数据、法线数据、纹理坐标等信息,你需要将这些数据传递给 WebGL 程序,以便在渲染循环中使用它们。
在 main.js
文件中添加以下代码:
// Assume that modelData is an object containing the data for the 3D model (vertices, normals, texture coordinates, etc.) const modelData = { /* ... */ };
6. 绘制3D图像
现在我们可以开始绘制3D图像了,将着色器程序设置为当前活动的程序,为每个顶点属性分配一个缓冲区对象,并将顶点数据上传到 WebGL,接下来,启用顶点数组对象(VAO)以管理顶点属性的状态,在渲染循环中绘制3D图像。
在 main.js
文件中添加以下代码:
// Set up the shader program and buffers for the 3D image function setupBuffers() { // ... (Set up the shader program and buffers for the 3D image) ... }
在渲染循环中调用 setupBuffers()
函数:
function render() { requestAnimationFrame(render); // Request the next animation frame and call render() when it's available render(); // Call render() immediately to start the animation loop (this will be called again by requestAnimationFrame()) } render(); // Start the animation loop by calling render() once at the beginning of the script execution (this will also call render() immediately)
7. 优化和调试 WebGL 应用程序