The simple graphical editor deals with a rectangular table M × N (1 ≤ M, N ≤ 250). Each pixel of the table has its colour. The picture is formed from this square pixels.
The problem is to write a program, which simulates an interactive work of the graphical editor.
Input consists of the editor commands, one per line. Each command is represented by one Latin capital placed in the very beginning of the line. If the command supposes parameters, all the parameters will be given in the same line separated by space. As the parameters there may be: the coordinates of the pixel - two integers, the first one is the column number and belongs to 1 … M, the second one is the row number and belongs to 1 … N, the origin is in the upper left corner of the table; the colour - the Latin capital; file name - in MSDOS 8.3 format.
The editor deals with the following commands:
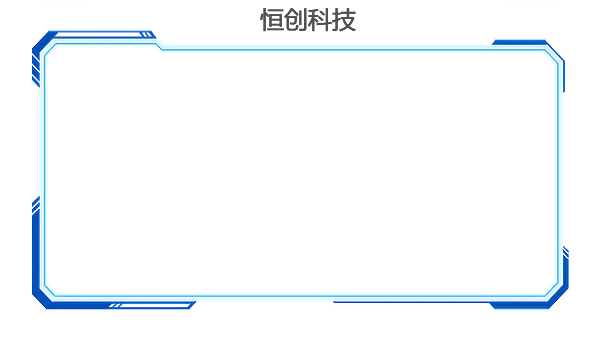
Every time the command ‘S NAME’ meets, you should output the file name NAME and the current table, row by row. Each row is represented by a pixels’ colours series, see the output sample.
Errors: If as a command there will be a character different from I, C, L, V, H, K, F, S, X, the editor should ignore the whole line and pass to the next command.
In case of other errors the program behaviour is unpredictable.
I 5 6
L 2 3 A
S one.bmp
G 2 3 J
F 3 3 J
V 2 3 4 W
H 3 4 2 Z
S two.bmp
X
one.bmp
OOOOO
OOOOO
OAOOO
OOOOO
OOOOO
OOOOO
two.bmp
JJJJJ
JJZZJ
JWJJJ
JWJJJ
JJJJJ
JJJJJ
题意:按照表格中给出的命令和命令所需的参数编写一个模拟图像编辑器的程序。
思路:前六条的命令比较简单,可以直接编写,但是要注意的是给出的参数不一定是按照从小到大的顺序,所以得到所需的参数后还需要稍作调整,这里是一个陷阱;第八条就是修改这个图像的名称后输出所有的像素的颜色,最后一条命令就是结束这个模拟程序;
最大的难点就在第七条命令,改变一个区域的颜色,这个区域满足的条件是,若(X,Y)属于这个区域,那么与这个像素相邻的像素点(必须要有公共边,每个像素点最多只有4个相邻的像素点)且颜色也相同的像素点也属于这个区域;所有我们可以使用递归进行深度遍历,改变同一个区域的颜色,这里有一个陷阱要注意的是,当要改变的颜色和这个区域本身的颜色是相同的,会陷入死循环,所以在这之前首先需要判断一下需要修改的颜色。
#include <stdio.h>
char image[255][255];//image
int row, col;//row x col image
void clearImage(){
for(int i = 1; i <= row; i++)
for(int j = 1; j <=col; j++)
image[i][j] = 'O';
}//command of C
void createImage(){
scanf("%d %d", &col, &row);
clearImage();
}//command of I M N
void printImage(char *name){
printf("%s\n", name);
for(int i = 1; i <= row; i++){
for(int j = 1; j <= col; j++)
putchar(image[i][j]);
putchar('\n');
}
}
void colorImage(){
int x, y;
char color;
scanf("%d %d %c", &x, &y, &color);
image[y][x] = color;
}//command of L X Y C
void colorVerImage(){
int x, y1, y2;
char color;
scanf("%d %d %d %c", &x, &y1, &y2, &color);
if(y1 > y2){
int temp = y1;
y1 = y2;
y2 = temp;
}
for(int i = y1; i <= y2; i++)
image[i][x] = color;
}//command of V X Y1 Y2 C
void colorHorImage(){
int x1, x2, y;
char color;
scanf("%d %d %d %c", &x1, &x2, &y, &color);
if(x1 > x2){
int temp = x1;
x1 = x2;
x2 = temp;
}
for(int i = x1; i <= x2; i++)
image[y][i] = color;
}//command of H X1 X2 Y C
void colorRecImage(){
int x1, x2, y1, y2;
char color;
scanf("%d %d %d %d %c", &x1, &y1, &x2, &y2, &color);
if(x1 > x2){
int temp = x1;
x1 = x2;
x2 = temp;
}
if(y1 > y2){
int temp = y1;
y1 = y2;
y2 = temp;
}
for(int i = y1; i <= y2; i++)
for(int j = x1; j <= x2; j++)
image[i][j] = color;
}//command of K X1 Y1 X2 Y2 C
void colorRegion(int x, int y, char newColor, char oldColor){
if(image[x][y] == oldColor){
image[x][y] = newColor;
if(x-1 > 0)
colorRegion(x-1, y, newColor, oldColor);
if(x+1 <= row)
colorRegion(x+1, y, newColor, oldColor);
if(y+1 <= col)
colorRegion(x, y+1, newColor, oldColor);
if(y-1 > 0)
colorRegion(x, y-1, newColor, oldColor);
}
}
void colorRegImage(){
int x, y;
char color, oldColor;
scanf("%d %d %c", &x, &y, &color);
oldColor = image[y][x];
if(oldColor == color)
return;
if(y >= 1 && y <= row && x >=1 && x <= col)
colorRegion(y, x, color, oldColor);
}//command of F X Y C
int main()
{
char com;//command
bool running = true;
bool ifget = true;
char name[50];//file name
while(1){
scanf("%c", &com);
switch(com){
case 'I':
createImage();
break;
case 'C':
clearImage();
break;
case 'L':
colorImage();
break;
case 'V':
colorVerImage();
break;
case 'H':
colorHorImage();
break;
case 'K':
colorRecImage();
break;
case 'F':
colorRegImage();
break;
case 'S':
scanf("%s", name);
printImage(name);
break;
case 'X':
running = false;
break;
default:
char temp[100];
gets(temp);
ifget = false;
break;
}
if(ifget)
getchar();
ifget = true;
if(!running)
break;
}
return 0;
}